In this post I am going to implement a quick search in SharePoint list using JQuery that allows you to search records in a list. It is useful in a situation where you don’t have SharePoint Search setup and just need a simple way of performing search operations in a SharePoint List.
To outline the solution please follow the below steps –
- Download the latest JQuery file from Here
- Create a Custom SharePoint list in which you would like to implement the search
- Open the SharePoint Designer
- Go to All Files-> Lists->Select the name of the list we just created
- Paste the JQuery file (jquery-min.js) we just downloaded
- Create a new JavaScript File-> Rename the file as “QuickSearch.js”
- Open “AllItem.aspx” view and add the reference of both the JQuery file
<script type=”text/javascript” src=” QuickSearch.js”>
<script type=”text/javascript” src=”jquery-min.js”>
- Now Edit the “QuickSearch.js” file, type below code and Save the file
$(document).ready(function(){
$(“input.search”).change(function() {
var txt = $(“input.search”).val();
if (txt) {
$(“#WebPartWPQ1”).find(“tr.ms-itmhover:not(:Contains(“+txt+”))”).hide();
$(“#WebPartWPQ1”).find(“tr.ms-itmhover:Contains(“+txt+”)”).show();
} else {
$(“#WebPartWPQ1”).find(“tr.ms-itmhover”).show();
}
}).keyup(function(){$(this).change();
});
});
<div style=”padding: 5px; border: 1px solid #cccccc; width: 60%; margin: 10px auto 0pt; background: none repeat scroll 0% 0% #efefef; display: block;”>
<h3 style=”text-align: center;”>TYPE HERE : <input class=”search” style=”padding: 10px;” type=”text”/>
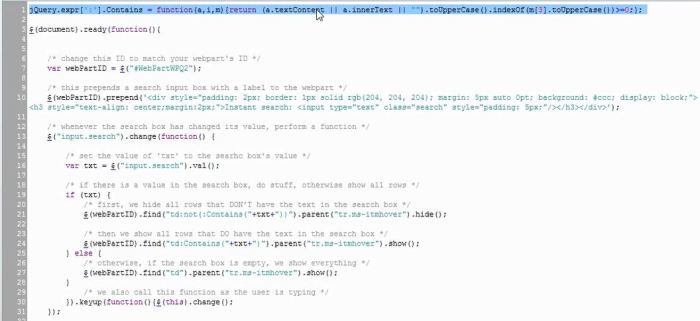
Explanation : We would like to start our function anytime the search input text is changed. Once it’s changed, it sets the value of a variable we have called “txt”.
Here we have an IF statement that just checks if there is indeed something in the search box, ELSE everything goes back to normal. If there is something inside the search input box, we are going to do two things. First, we’ll find any row that does NOT contain the string, and we’ll hide that row. Then we’ll find any row that DOES contain that string, and we’ll show that row. Finally, we chain a “keyup” function to the change function so that as we type everything is happening all together.
And last but not least, we created a simple search input box with a class of “search”.
Now open the SharePoint List All Item view in internet explorer and If you have done everything correctly then it will filter the items on the basis of your inputs.
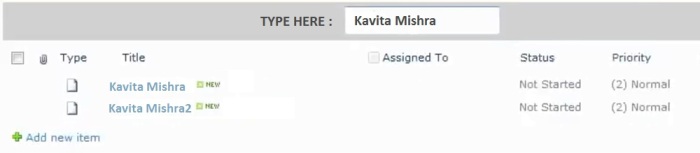
PS : You’ll have to change the target WebPart ID it’s searching in and replace it with your current Web Part ID and its row class.
I hope you find this post useful.